Blazor 8 InteractiveAuto & Authentication: navigating the pitfalls
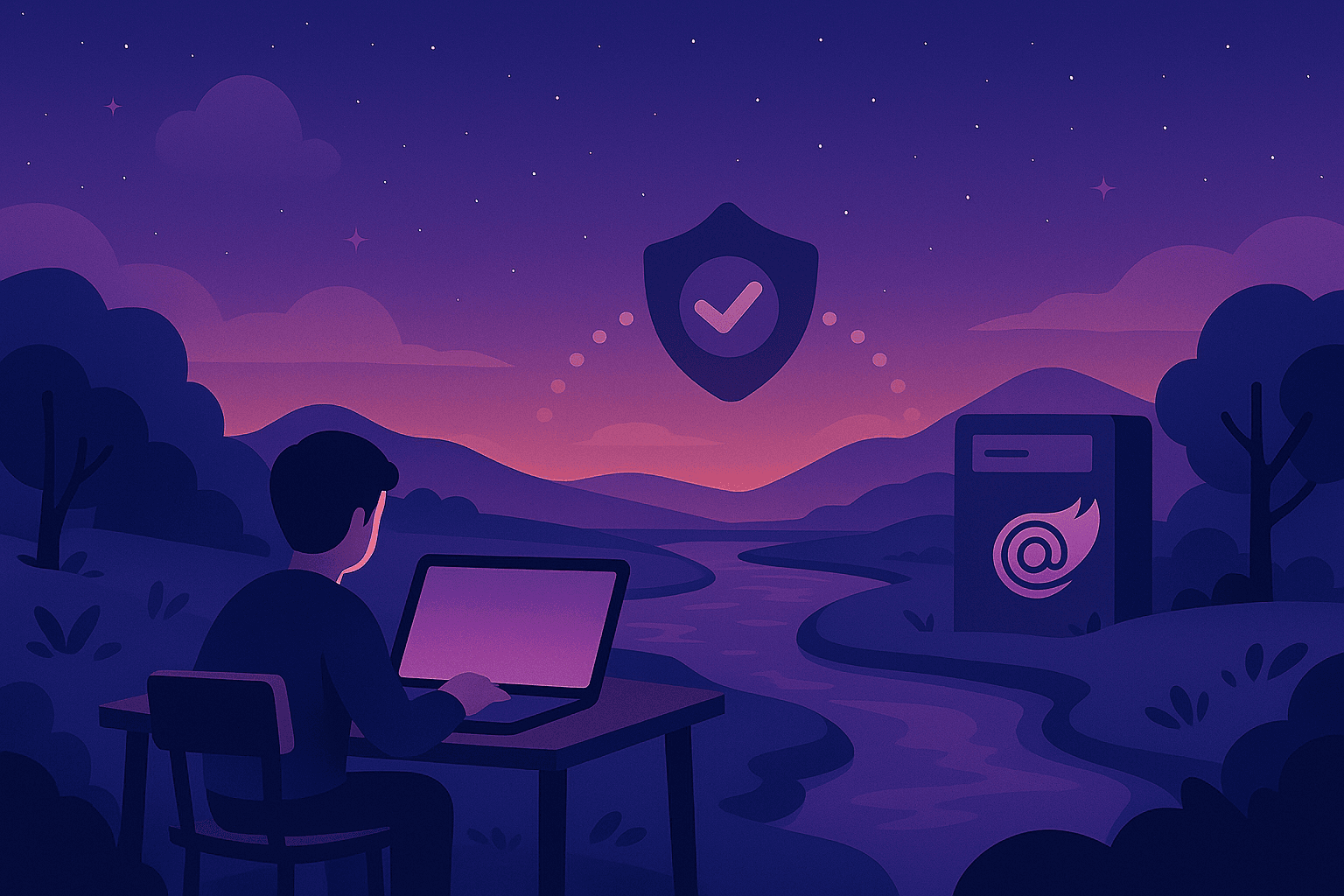
Blazor 8's new render mode, InteractiveAuto, promises server-side speed on first load and WebAssembly (WASM) efficiency for subsequent interactions. Sounds ideal, right? Well, yes—if you manage to avoid the authentication headaches it can introduce.
Having recently battled endless login redirects, duplicate API calls, and plenty of frustration, I've distilled some critical insights that could save you considerable debugging hours.
Quick Overview of Blazor Render Modes
Here's a practical breakdown of what each Blazor render mode means for authentication:
Mode | Server-side Prerender | Client Execution | Auth Type | Pros / Cons |
---|---|---|---|---|
Static | ✅ | ❌ | None | 🟢 Instant load, 🔴 Zero interactivity |
InteractiveServer | ✅ | ✅ (SignalR) | Cookie (Session) | 🟢 SEO-friendly, 🔴 Heavy resource use |
InteractiveWebAssembly (prerender) | ✅ | ✅ | Token (Bearer) | 🟢 No SignalR overhead, 🔴 Double-render quirks |
InteractiveWebAssembly (no prerender) | ❌ | ✅ | Token (Bearer) | 🟢 Single clean render, 🔴 Initial blank screen delay |
InteractiveAuto | ✅ (initial) | ✅ (WASM later) | Cookie ➜ Token | 🟢 Fast first paint, smooth SPA later; 🔴 Auth complexities |
Note: InteractiveAuto never dynamically switches mode mid-render—it transitions fully upon navigation or refresh.
Why Authentication Is Messy in InteractiveAuto
The Double Render Dilemma
Your initialization methods (OnInitializedAsync
) run twice:
- Server-side prerender: Authenticated via cookie, returning valid data.
- WASM execution: Lacks immediate token access, resulting in repeated requests and potential 401 errors.
Result: Confusion, wasted resources, and suboptimal user experience.
Cookies vs Tokens: An Awkward Partnership
- Cookies are automatic and secure (if HttpOnly) but limited to same-origin calls.
- Bearer Tokens are flexible for APIs, but require careful client-side management (XSS protection, secure storage).
InteractiveAuto awkwardly bridges these two authentication methods, demanding a precise handoff from cookie-based auth to token-based auth.
Effective Strategies for Smooth Authentication
1. Cache Results from the Server-Side Render
Minimize redundant API calls by caching the server response:
public class UserProfileService
{
private UserDto? _cached;
private readonly HttpClient _http;
public UserProfileService(HttpClient http) => _http = http;
public async Task<UserDto?> GetAsync()
{
if (_cached is not null) return _cached;
_cached = await _http.GetFromJsonAsync<UserDto>("api/user");
return _cached;
}
}
Register this as Scoped
to reuse across renders.
2. Proper Token Handoff (Cookie ➜ Token)
Request and inject tokens immediately after initial rendering:
@code {
[Inject] IAccessTokenProvider TokenProvider { get; set; } = default!;
protected override async Task OnAfterRenderAsync(bool firstRender)
{
if (firstRender)
{
var result = await TokenProvider.RequestAccessToken();
if (result.TryGetToken(out var token))
{
await JS.InvokeVoidAsync("auth.setToken", token.Value);
}
}
}
}
JavaScript handler (auth.js
):
window.auth = {
setToken: t => localStorage.setItem('access_token', t),
getToken: () => localStorage.getItem('access_token')
};
3. Keep API and UI Separate
Combining UI and API in one server can result in confusing responses (like HTML login pages where JSON is expected). Isolate your API endpoints to maintain clear, predictable responses.
4. Use a Proxy (YARP)
Employ YARP to consistently handle token forwarding and proxying:
// Configure YARP
// Token forwarding example
transformBuilderContext.AddRequestTransform(async ctx =>
{
var token = await ctx.HttpContext.GetTokenAsync("access_token");
if (!string.IsNullOrEmpty(token))
{
ctx.ProxyRequest.Headers.Authorization = new("Bearer", token);
}
});
Tip: This ensures tokens are always correctly attached, minimizing auth failures.
Practical Client-Side Setup
Here's a streamlined client-side initialization for clarity:
var builder = WebAssemblyHostBuilder.CreateDefault(args);
builder.Services.AddAuthorizationCore();
builder.Services.AddCascadingAuthenticationState();
builder.Services.AddAuthenticationStateDeserialization();
builder.Services.AddHttpClient<IPublishyClient>(client =>
client.BaseAddress = new(builder.HostEnvironment.BaseAddress));
builder.Services.AddScoped<IPublishyClient, PublishyClient>();
await builder.Build().RunAsync();
The AddAuthenticationStateDeserialization()
optimizes user state restoration after refresh.
The Verdict: Is InteractiveAuto Worth the Trouble?
✅ Best Practices:
- Cache prerendered data
- Inject tokens immediately
- Isolate API and UI concerns
- Use proxies effectively
❌ Avoid:
- Repeated API calls
- Late token discovery
- Combining UI/API logic confusingly
InteractiveAuto certainly adds complexity, especially compared to React’s more mature client-server patterns. It can deliver great performance, but achieving that smoothly requires deliberate effort and careful architecture.
Conclusion
Blazor 8’s InteractiveAuto mode offers significant benefits—if you're prepared to navigate its authentication challenges. Use the strategies above to streamline your experience, but always critically assess whether this complexity aligns with your project's actual needs. Sometimes, simpler (or React with Next !) might indeed be better.